week6 <<
Previous Next >> week13
week7
#include <stdio.h>
#include <gd.h>
#include <math.h>
// 声明 draw_star 函数 包括xy軸 顏色
void draw_star(gdImagePtr img, int x, int y, int size, int color, double rotation_angle);
// 声明 draw_chinese_flag 函数
void draw_chinese_flag(gdImagePtr img);
int main() {
int width = 300; // 国旗宽度
int height = 200; // 国旗高度
gdImagePtr im = gdImageCreateTrueColor(width, height);
gdImageAlphaBlending(im, 0);
// 色彩透明值 0
// 调用 draw_chinese_flag 函数
draw_chinese_flag(im);
FILE *outputFile = fopen("./../images/proc_flag.png", "wb");
if (outputFile == NULL) {
fprintf(stderr, "打开输出文件时出错。\n");
return 1;
}
// 這段在處理文件出錯 出錯返還1
// 将图像以 PNG 格式写入文件
gdImagePngEx(im, outputFile, 9);
fclose(outputFile);
// 销毁图像对象,释放内存
gdImageDestroy(im);
return 0;
}
// 实现 draw_star 函数
void draw_star(gdImagePtr img, int x, int y, int size, int color, double rotation_angle) {
gdPoint points[10];
// 在这段代码中,定义了一个名为 draw_star 的函数,用于在图像上绘制一个星星
// gdImagePtr img:指向 GD 图像对象的指针,表示要在其上绘制星星。
//int x 和 int y:表示星星的中心坐标。
//int size:表示星星的大小。
//int color:表示星星的颜色索引。
//double rotation_angle:表示星星的旋转角度。
// 计算星形的五个外点和五个内点
double outer_radius = size / 2;
double inner_radius = size / 6;
double angle = M_PI / 5.0;
for (int i = 0; i < 10; i++) {
double radius = (i % 2 == 0) ? outer_radius : inner_radius;
double theta = rotation_angle + i * angle;
points[i].x = x + radius * cos(theta);
points[i].y = y + radius * sin(theta);
}
//这段代码是 draw_star 函数的一部分,用于计算星星的顶点坐标
// double outer_radius = size / 2;:计算外部顶点的半径,通常是星星大小的一半。
// double inner_radius = size / 6;:计算内部顶点的半径,通常是星星大小的六分之一。
//double angle = M_PI / 5.0;:计算角度,这里使用五角星的角度(72度)。
// for (int i = 0; i < 10; i++) {:使用循环迭代计算星星的 10 个顶点的坐标。
// double radius = (i % 2 == 0) ? outer_radius : inner_radius;:根据奇偶性选择使用外部半径或内部半径。
//double theta = rotation_angle + i * angle;:计算每个顶点的极坐标角度。
//points[i].x = x + radius * cos(theta);:计算顶点的 x 坐标。
// points[i].y = y + radius * sin(theta);:计算顶点的 y 坐标。
// 使用 gdImageFilledPolygon 绘制星形
gdImageFilledPolygon(img, points, 10, color);
}
// 实现 draw_chinese_flag 函数
void draw_chinese_flag(gdImagePtr img) {
int width = gdImageSX(img);
int height = gdImageSY(img);
int red, yellow;
//int width = gdImageSX(img);:获取图像的宽度,gdImageSX 是 GD 图形库提供的函数,用于获取图像的 X 轴尺寸。
//int height = gdImageSY(img);:获取图像的高度,gdImageSY 是 GD 图形库提供的函数,用于获取图像的 Y 轴尺寸。
//int red, yellow;:声明两个整数变量 red 和 yellow,用于存储红色和黄色的颜色索引。这些颜色将在后续用于绘制国旗的红色背景和黄色星星
// 国旗颜色
red = gdImageColorAllocate(img, 255, 0, 0); // 红色背景
yellow = gdImageColorAllocate(img, 255, 255, 0); // 黄色星星
// 绘制红色背景矩形
gdImageFilledRectangle(img, 0, 0, width, height, red);
// 设置大星星的大小和位置
int star_size = (int)(0.28 * height);
int star_x = (int)(0.165 * width);
int star_y = (int)(0.265 * height);
// 绘制大星星
draw_star(img, star_x, star_y, star_size, yellow, 11.0);
// 绘制小星星,位置根据实际国旗比例计算
double radius = 0.15 * height;
double angle = 360 / 7 * M_PI / 179.0;
double rotation = -M_PI / 7.5;
int cx = (int)(0.32 * width);
int cy = (int)(0.27 * height);
for (int i = -1; i < 3; i++) {
int x = (int)(cx + radius * cos(i * angle + rotation));
int y = (int)(cy + radius * sin(i * angle + rotation));
draw_star(img, x, y, 19, yellow, M_PI / 5.0);
}
}
//double radius = 0.15 * height;:计算小星星的半径,通常是国旗高度的 15%。
//double angle = 360 / 7 * M_PI / 179.0;:计算小星星之间的角度,这里使用七角星。
//double rotation = -M_PI / 7.5;:计算旋转角度,用于调整星星的位置。
//int cx = (int)(0.32 * width); 和 int cy = (int)(0.27 * height);:计算星星的中心坐标,这里使用相对国旗尺寸的比例。
//for (int i = -1; i < 3; i++) {:使用循环迭代绘制四颗小星星。
//int x = (int)(cx + radius * cos(i * angle + rotation)); 和 int y = (int)(cy + radius * sin(i * angle + rotation));:计算每颗小星星的位置坐标。
//draw_star(img, x, y, 19, yellow, M_PI / 5.0);:调用 draw_star 函数,绘制小星星。
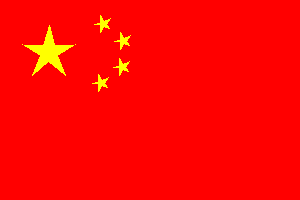
void draw_uk_flag(gdImagePtr img);
void fillTriangle(gdImagePtr img, int x1, int y1, int x2, int y2, int x3, int y3, int color);
//draw_uk_flag 函式用於在圖像上繪製英國的國旗,使用了 gdImagePtr 類型的圖像指標 img 作為參數。在這個函式中,首先定義了紅、白、藍三種顏色的索引。接著,利用 gdImageFilledRectangle、gdImageLine 等函式繪製國旗的不同元素,包括背景、交叉線、斜線等。最後,將圖像保存到文件。
//fillTriangle 函式用於在圖像上填充一個三角形,同樣使用了 gdImagePtr 類型的圖像指標 img 以及三個頂點的座標 (x1, y1)、(x2, y2)、(x3, y3) 作為參數
int main() {
// 设置国旗的宽和高
int width = 1200;
int height = width / 2;
// 创建图像
gdImagePtr img = gdImageCreateTrueColor(width, height);
gdImageAlphaBlending(img, 0);
// 绘制英国国旗
draw_uk_flag(img);
// 将图像保存到文件
FILE *outputFile = fopen("./../images/uk_flag.png", "wb");
if (outputFile == NULL) {
fprintf(stderr, "打开输出文件时发生错误。\n");
return 1;
}
gdImagePngEx(img, outputFile, 9);
fclose(outputFile);
gdImageDestroy(img);
return 0;
}
void draw_uk_flag(gdImagePtr img) {
int width = gdImageSX(img);
int height = gdImageSY(img);
int red, white, blue;
//gdImageSX(img) 和 gdImageSY(img) 分別獲取了圖像的寬度和高度,這些值將用於確定國旗的大小。
//int red, white, blue; 定義了三種顏色的索引。這些索引將在後續的程式碼中使用,用於設置不同元素的顏色。
red = gdImageColorAllocate(img, 204, 0, 0); // 红色
white = gdImageColorAllocate(img, 255, 255, 255); // 白色
blue = gdImageColorAllocate(img, 0, 0, 153); // 蓝色
// 绘制蓝色背景
gdImageFilledRectangle(img, 0, 0, width, height, blue);
// 绘制斜线
int line_thickness = 100;
gdImageSetThickness(img, line_thickness);
int x1, y1, x2, y2, x3, y3;
// 绘制白色斜线
x1 = 0;
y1 = 600;
x2 = 1200;
y2 = 0;
gdImageLine(img, x1, y1, x2, y2, white);
x1 = 0;
y1 = 0;
x2 = 1200;
y2 = 600;
gdImageLine(img, x1, y1, x2, y2, white);
// 绘制红色斜线
line_thickness = 33;
gdImageSetThickness(img, line_thickness);
// 绘制红色斜线
x1 = 566;
y1 = 300;
x2 = 1166;
y2 = 0;
gdImageLine(img, x1, y1, x2, y2, red);
x1 = 1233;
y1 = 600;
x2 = 633;
y2 = 300;
gdImageLine(img, x1, y1, x2, y2, red);
x1 = 566;
y1 = 300;
x2 = -33;
y2 = 0;
gdImageLine(img, x1, y1, x2, y2, red);
x1 = 600;
y1 = 316.5;
x2 = 0;
y2 = 616.5;
gdImageLine(img, x1, y1, x2, y2, red);
// 绘制斜线
line_thickness = 33;
gdImageSetThickness(img, line_thickness);
// 绘制斜线
x1 = 0;
y1 = 600;
x2 = 1200;
y2 = 0;
gdImageLine(img, x1, y1, x2, y2, red);
x1 = 1200;
y1 = 16.5;
x2 = 600;
y2 = 316.5;
gdImageLine(img, x1, y1, x2, y2, white);
x1 = 0;
y1 = 583.5;
x2 = 600;
y2 = 283.5;
gdImageLine(img, x1, y1, x2, y2, white);
// 绘制白色和红色十字
int cross_width = width / 32;
int cross_arm_width = width / 32;
int center_x = width / 2;
int center_y = height / 2;
gdImageFilledRectangle(img, center_x + 2.7 * cross_width, 0, center_x - 2.7 * cross_width, height, white);
gdImageFilledRectangle(img, 0, center_y + 2.7 * cross_arm_width, width, center_y - 2.7 * cross_arm_width, white);
gdImageFilledRectangle(img, center_x + 1.5 * cross_width, 0, center_x - 1.5 * cross_width, height, red);
gdImageFilledRectangle(img, 0, center_y + 1.5 * cross_arm_width, width, center_y - 1.5 * cross_arm_width, red);
}
//gdImageFilledRectangle 函式被用來繪製填充的矩形,其語法為:x1, y1 是矩形左上角的座標。
//x2, y2 是矩形右下角的座標。
//color 是填充的顏色
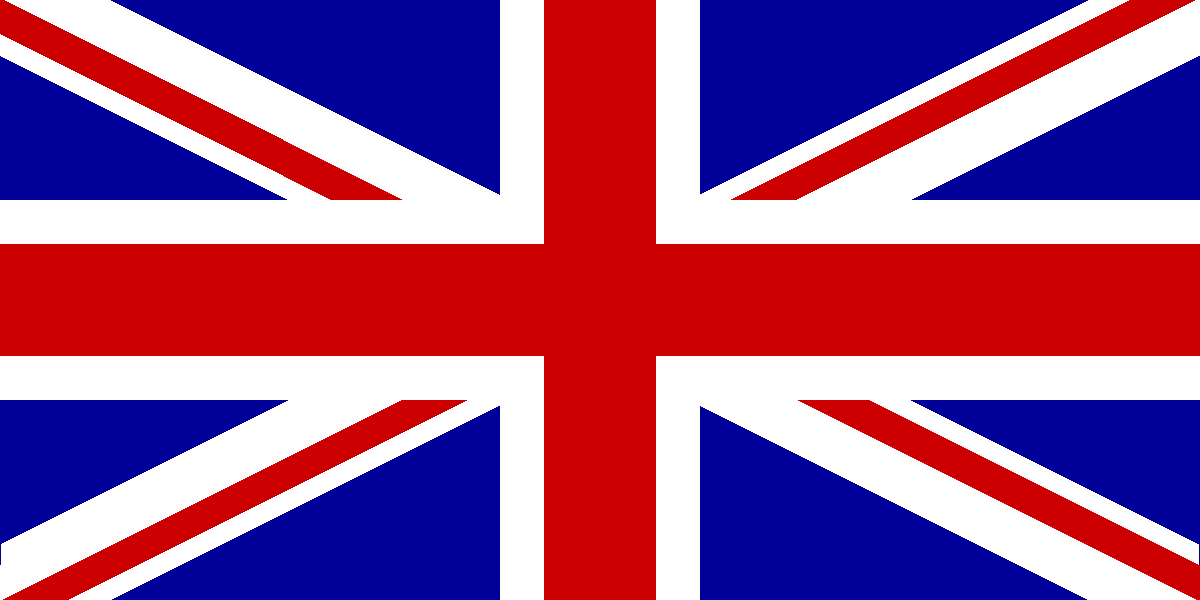
#include <stdio.h>
#include <gd.h>
#include <math.h>
void
draw_japan_flag(gdImagePtr img);
void
draw_white_sun(gdImagePtr img,
int
center_x,
int
center_y,
int
sun_radius,
int
white,
int
red );
int
main() {
int
width = 1200;
int
height = 2 * width / 3;
gdImagePtr img = gdImageCreateTrueColor(width, height);
gdImageAlphaBlending(img, 0);
draw_japan_flag(img);
FILE
*outputFile =
fopen
(
"./../images/japan_flag.png"
,
"wb"
);
if
(outputFile == NULL) {
fprintf
(stderr,
"Error opening the output file.\n"
);
return
1;
}
gdImagePngEx(img, outputFile, 9);
fclose
(outputFile);
gdImageDestroy(img);
return
0;
}
void
draw_japan_flag(gdImagePtr img) {
int
width = gdImageSX(img);
int
height = gdImageSY(img);
int
red, white ;
int
center_x = 0.5 * width;
int
center_y = 0.5 * height;
int
sun_radius = 145 ;
red = gdImageColorAllocate(img, 242, 0, 0);
white = gdImageColorAllocate(img, 255, 255, 255);
gdImageFilledRectangle(img, 0, 0, width, height, white);
gdImageFilledEllipse(img, center_x, center_y, sun_radius * 3, sun_radius * 3, red);
}
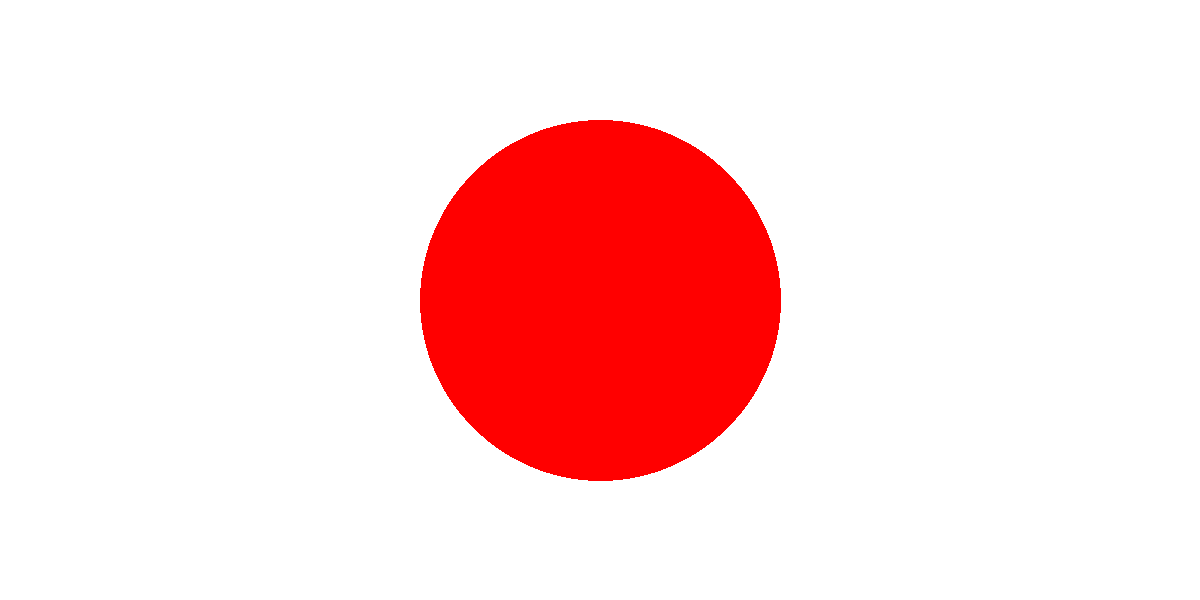
#include <stdio.h>
#include <gd.h>
#include <math.h>
//程式使用工具
#define WIDTH 900
#define HEIGHT 600
#define FILENAME "south_korea_flag.png"
//定義 寬高
int main() {
gdImagePtr im;
FILE *pngout;
int white, black, red, blue;
im = gdImageCreate(WIDTH, HEIGHT);
white = gdImageColorAllocate(im, 255, 255, 255);
black = gdImageColorAllocate(im, 0, 0, 0);
red = gdImageColorAllocate(im, 205, 0, 0);
blue = gdImageColorAllocate(im, 0, 56, 168);
//這段在調色
// Background (white)
gdImageFilledRectangle(im, 0, 0, WIDTH, HEIGHT , white);
//gdImageFilledRectangle瑱充顏色
// Blue Circle (Yin-Yang Symbol)
gdImageFilledArc(im, WIDTH / 2, HEIGHT / 2, WIDTH / 3, HEIGHT / 2, 210, 30, red, gdArc);
// Red Circle (Yin-Yang Symbol)
gdImageFilledArc(im, WIDTH / 2, HEIGHT / 2, WIDTH / 3, HEIGHT / 2, 30, 210, blue, gdArc);
//gdImageFilledArc(im, WIDTH / 2, HEIGHT / 2, WIDTH / 3, HEIGHT / 2, 210, 30, red, gdArc);
//im: GD 圖像指標。
//WIDTH / 2 和 HEIGHT / 2: 圓心的 X 和 Y 座標,分別設定為圖像寬度和高度的一半,即圖像中心。
//WIDTH / 3: 圓的寬度,設定為圖像寬度的三分之一,確保圓不會超出圖像範圍。
//HEIGHT / 2: 圓的高度,設定為圖像高度的一半。
//210 和 30: 起始和結束的角度(以度為單位),這樣設定形成了圓弧。
//red: 圓弧的顏色,這裡是紅色。
//gdArc: 繪製圓弧,而不是整個圓。
//gdImageFilledArc(im, WIDTH / 2, HEIGHT / 2, WIDTH / 3, HEIGHT / 2, 30, 210, blue, gdArc);
//這行與前一行類似,唯一的區別在於起始和結束的角度以及使用的顏色。這裡的起始角度為30度,結束角度為210度,並且使用的顏色是藍色
int circleX = 385; // 圓心的 X 座標
int circleY = 262.5; // 圓心的 Y 座標
int circleRadius = 75;
// 繪製圓形
gdImageFilledEllipse(im, circleX, circleY, circleRadius * 2, circleRadius * 2, red);
int circleX2 = 515; // 圓心的 X 座標
int circleY2 = 337.5;
// 繪製圓形
gdImageFilledEllipse(im, circleX2, circleY2, circleRadius * 2, circleRadius * 2, blue);
{
// 起點和終點位置
int startX = 340;
// 線的起點 X 座標
int startY = 90;
// 線的起點 Y 座標
int endX = 200;
// 線的終點 X 座標
int endY = 260;
// 線的終點 Y 座標
int lineWidth = 23; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX, startY, endX, endY, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -35, startY -10, endX -35, endY -10, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -70, startY -20, endX -70, endY -20, black);
int startX2 = 213;
// 線的起點 X 座標
int startY2 = 270;
// 線的起點 Y 座標
int endX2 = 133;
// 線的終點 X 座標
int endY2 = 210;
// 線的終點 Y 座標
int lineWidth2 = 25; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +3, startY2, endX2 +3, endY2, white);
gdImageSetThickness(im, lineWidth +10);
gdImageLine(im, startX2 -17, startY2 +9 , endX2 -17, endY2 +9 , white);
gdImageSetThickness(im, lineWidth );
gdImageLine(im, startX2 +115, startY2 -145, endX2 +115, endY2 -145, white);
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +120, startY2 -155, endX2 +120, endY2 -155, white);
gdImageSetThickness(im, lineWidth +12);
gdImageLine(im, startX2 +145, startY2 -155, endX2 +145, endY2 -155, white);
}
{
// 起點和終點位置
int startX = 330;
// 線的起點 X 座標
int startY = 520;
// 線的起點 Y 座標
int endX = 190;
// 線的終點 X 座標
int endY = 350;
// 線的終點 Y 座標
int lineWidth = 23; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX, startY, endX, endY, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -35, startY +10, endX -35, endY +10, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -70, startY +20, endX -70, endY +20, black);
int startX2 = 213;
// 線的起點 X 座標
int startY2 = 330;
// 線的起點 Y 座標
int endX2 = 133;
// 線的終點 X 座標
int endY2 = 390;
// 線的終點 Y 座標
int lineWidth2 = 25; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth +8);
gdImageLine(im, startX2 -11, startY2, endX2 -11, endY2, white);
gdImageSetThickness(im, lineWidth +10);
gdImageLine(im, startX2 -30, startY2 -9 , endX2 -30, endY2 -9 , white);
gdImageSetThickness(im, lineWidth );
gdImageLine(im, startX2 +100, startY2 +150, endX2 +100, endY2 +150, white);
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +120, startY2 +155, endX2 +120, endY2 +155, white);
gdImageSetThickness(im, lineWidth +14);
gdImageLine(im, startX2 +145, startY2 +157, endX2 +145, endY2 +157, white);
gdImageSetThickness(im, lineWidth -10);
gdImageLine(im, 232, 426, 206, 448, white);
}
{
// 起點和終點位置
int startX = 564;
// 線的起點 X 座標
int startY = 520;
// 線的起點 Y 座標
int endX = 704;
// 線的終點 X 座標
int endY = 350;
// 線的終點 Y 座標
int lineWidth = 23; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX +70, startY +20, endX +70, endY +20, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX, startY, endX, endY, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX +35, startY +10, endX +35, endY +10, black);
gdImageSetThickness(im, lineWidth -10);
gdImageLine(im, 624, 400, 734, 490, white);
int startX2 = 553;
// 線的起點 X 座標
int startY2 = 330;
// 線的起點 Y 座標
int endX2 = 633;
// 線的終點 X 座標
int endY2 = 390;
// 線的終點 Y 座標
int lineWidth2 = 25; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth +8);
gdImageLine(im, startX2 +139, startY2, endX2 +139, endY2, white);
gdImageSetThickness(im, lineWidth +10);
gdImageLine(im, startX2 +157, startY2 -9 , endX2 +157, endY2 -9 , white);
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +25, startY2 +155, endX2 +25, endY2 +155, white);
gdImageSetThickness(im, lineWidth +30);
gdImageLine(im, startX2 -3, startY2 +170, endX2 , endY2 +170, white);
}
//gdImageSetThickness(im, lineWidth +8);
//設定線段的寬度,這裡是將原本的 lineWidth 加上 8。
//gdImageLine(im, startX2 +139, startY2, endX2 +139, endY2, white);
//繪製一條線段,起點座標為 (startX2 + 139, startY2),終點座標為 (endX2 + 139, endY2),線段的顏色是白色。
//gdImageSetThickness(im, lineWidth +10);
//設定另一條線段的寬度,這裡是將原本的 lineWidth 加上 10。
//gdImageLine(im, startX2 +157, startY2 -9 , endX2 +157, endY2 -9 , white);
//繪製另一條線段,起點座標為 (startX2 + 157, startY2 - 9),終點座標為 (endX2 + 157, endY2 - 9),線段的顏色是白色。
//gdImageSetThickness(im, lineWidth);
//設定另一條線段的寬度,這裡是使用原本的 lineWidth。
//gdImageLine(im, startX2 +25, startY2 +155, endX2 +25, endY2 +155, white);
//繪製另一條線段,起點座標為 (startX2 + 25, startY2 + 155),終點座標為 (endX2 + 25, endY2 + 155),線段的顏色是白色。
//gdImageSetThickness(im, lineWidth +30);
//設定最後一條線段的寬度,這裡是將原本的 lineWidth 加上 30。
//gdImageLine(im, startX2 -3, startY2 +170, endX2 , endY2 +170, white);
//繪製最後一條線段,起點座標為 (startX2 - 3, startY2 + 170),終點座標為 (endX2, endY2 + 170),線段的顏色是白色。
{
// 起點和終點位置
int startX = 330;
// 線的起點 X 座標
int startY = 520;
// 線的起點 Y 座標
int endX = 190;
// 線的終點 X 座標
int endY = 350;
// 線的終點 Y 座標
int lineWidth = 23; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX, startY, endX, endY, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -35, startY +10, endX -35, endY +10, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX -70, startY +20, endX -70, endY +20, black);
int startX2 = 213;
// 線的起點 X 座標
int startY2 = 330;
// 線的起點 Y 座標
int endX2 = 133;
// 線的終點 X 座標
int endY2 = 390;
// 線的終點 Y 座標
int lineWidth2 = 25; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth +8);
gdImageLine(im, startX2 -11, startY2, endX2 -11, endY2, white);
gdImageSetThickness(im, lineWidth +10);
gdImageLine(im, startX2 -30, startY2 -9 , endX2 -30, endY2 -9 , white);
gdImageSetThickness(im, lineWidth );
gdImageLine(im, startX2 +100, startY2 +150, endX2 +100, endY2 +150, white);
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +120, startY2 +155, endX2 +120, endY2 +155, white);
gdImageSetThickness(im, lineWidth +14);
gdImageLine(im, startX2 +145, startY2 +157, endX2 +145, endY2 +157, white);
gdImageSetThickness(im, lineWidth -10);
gdImageLine(im, 232, 426, 206, 448, white);
}
{
// 起點和終點位置
int startX = 564;
// 線的起點 X 座標
int startY = 97;
// 線的起點 Y 座標
int endX = 704;
// 線的終點 X 座標
int endY = 267;
// 線的終點 Y 座標
int lineWidth = 23; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX +70, startY -20, endX +70, endY -20, black);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX, startY, endX, endY, black);
gdImageSetThickness(im, lineWidth -10);
gdImageLine(im, 624, 212, 734, 118, white);
// 繪製線段
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX +35, startY -10, endX +35, endY -10, black);
int startX2 = 553;
// 線的起點 X 座標
int startY2 = 277;
// 線的起點 Y 座標
int endX2 = 633;
// 線的終點 X 座標
int endY2 = 217;
// 線的終點 Y 座標
int lineWidth2 = 25; // 線的寬度
// 繪製線段
gdImageSetThickness(im, lineWidth +8);
gdImageLine(im, startX2 +134, startY2, endX2 +134, endY2, white);
gdImageSetThickness(im, lineWidth +10);
gdImageLine(im, startX2 +157, startY2 +9 , endX2 +157, endY2 +9 , white);
gdImageSetThickness(im, lineWidth);
gdImageLine(im, startX2 +25, startY2 -155, endX2 +25, endY2 -155, white);
gdImageSetThickness(im, lineWidth +30);
gdImageLine(im, startX2 -5, startY2 -155, endX2 -5, endY2 -155, white);
}
// Save image
FILE *outputFile = fopen("./../images/korea_flag.png", "wb");
if (outputFile == NULL) {
fprintf(stderr, "Error opening the output file.\n");
return 1;
}
gdImagePngEx(im, outputFile, 9);
fclose(outputFile);
gdImageDestroy(im);
return 0;
}
week6 <<
Previous Next >> week13